本文最后更新于:2024年5月7日 下午
频域乘法表现在空域中等效于卷积计算,但是计算量会大大降低,本文记录 OpenCV 实现频域操作图像的相关内容。
概述
-
图像处理一般分为空间域处理和频率域处理,空间域处理是直接对图像内的像素进行处理。频率域处理是先将图像变换到频率域,然后在频率域对图像进行处理,最后通过反变换将图像变为空间域。傅里叶变换可以将图像变换为频率域, 傅立叶反变换再将频率域变换为空间域。
-
在频域里,对于一幅图像,高频部分代表了图像的、纹理信息;低频部分则代表了图像的轮廓信息。如果图像受到的噪声恰好在某个特定的频率范围内,就可以使用滤波器来恢复原来的图像。因此傅里叶变换在图像处理中可以做到图像增强和去噪、图像分割之边缘检测、图像特征提取和压缩等。
空域卷积
- 理论推导
- 实现时利用 OpenCV 函数计算 DFT 变换,频域乘法与 IDFT 变换
实例演示
- 测试图像
-
转换为灰度图像作为测试图像
-
卷积核为 x 方向上的 Sobel 算子
- 示例代码
1 |
|
经过频域计算的卷积结果与空域结果有一点点出入,可能和量化有关
图像处理
- 将图像转换到频域空间信息没有丢失,但是可以在频域中对图像进行处理
- JPEG 图像压缩用的就是丢去频域中高频信息的方式实现图像压缩的
实例演示
- 此处示例一种简单的图像低通、高通滤波
1 |
|
参考资料
文章链接:
https://www.zywvvd.com/notes/study/image-processing/opencv/opencv-dft-conv/opencv-dft-conv/
“觉得不错的话,给点打赏吧 ୧(๑•̀⌄•́๑)૭”
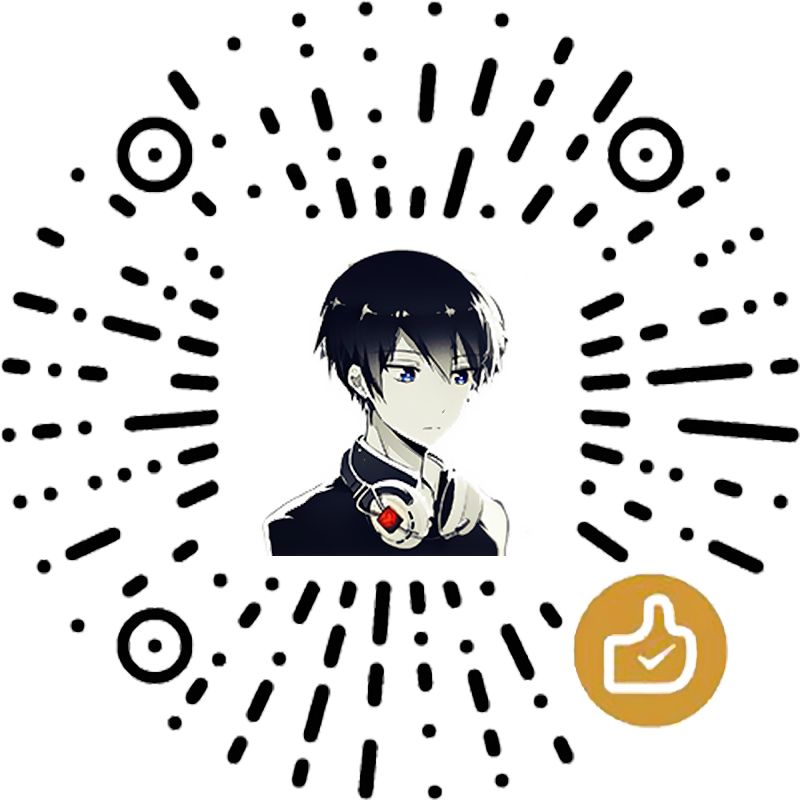
微信支付
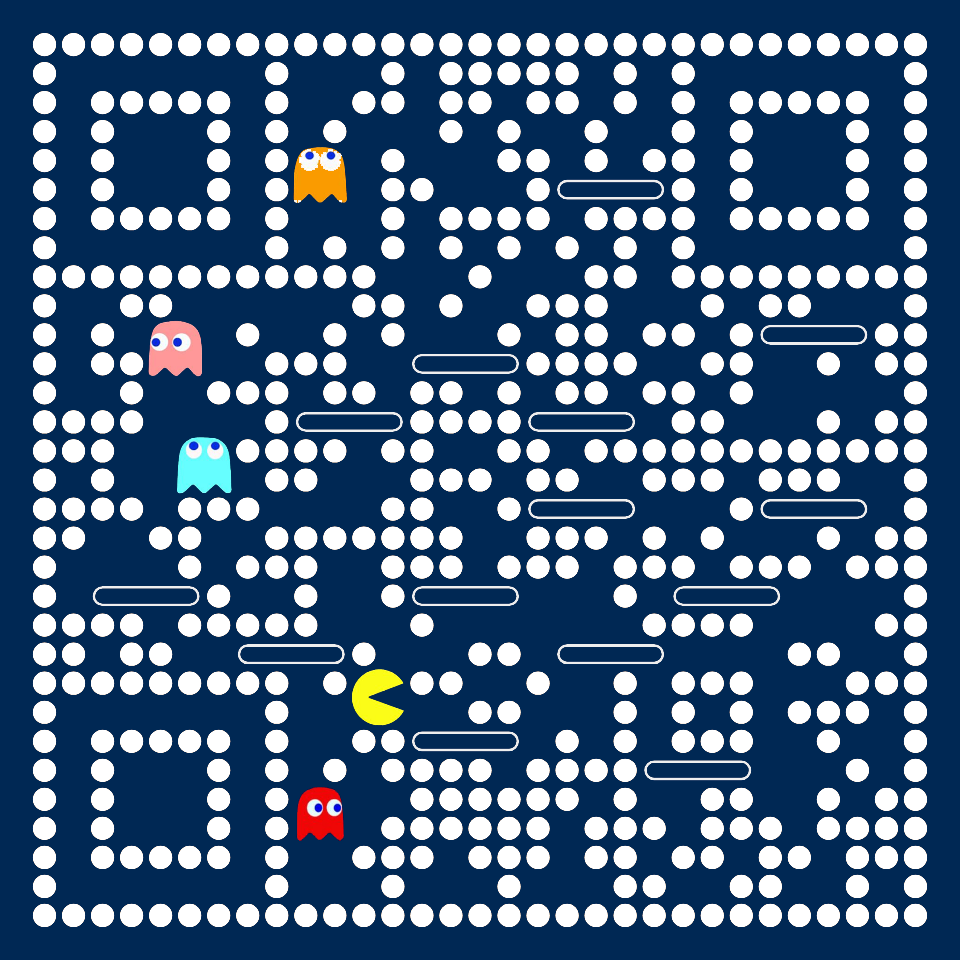
支付宝支付
OpenCV 图像频域操作
https://www.zywvvd.com/notes/study/image-processing/opencv/opencv-dft-conv/opencv-dft-conv/