本文最后更新于:2024年5月7日 下午
Next 主题使用swig引擎,swig 是node端的一个优秀简洁的模板引擎,本文介绍swig基本语法。
特性
- 支持大多数主流浏览器。
- 表达式兼容性好。
- 面向对象的模板继承。
- 将过滤器和转换应用到模板中的输出。
- 可根据路劲渲染页面。
- 支持页面复用。
- 支持动态页面。
- 可扩展、可定制。
安装
1 |
|
基本用法
swig有多种实现方式来编译和渲染模板
1 |
|
变量
模板中的变量使用双大括号来声明。例如
1 |
|
属性
变量的属性可以使用
.
或者[ ]
来访问,如下两个是等价的:
1 |
|
他遵循和javascript一样的原则,如果关键词中有
-
,则必须使用[]
来访问属性,不能使用.
Bad!
1 |
|
Good!
1 |
|
未定义和空值
如果一个变量未定义或者是空值,在渲染的时候,将会在相应的位置输出一个空的字符串,而不会报异常。
过滤器
变量可以用特殊的链式结构进行修改过滤。
1 |
|
方法(函数)
变量也可以是 JavaScript functions. 值得注意的是,不管你的 autoescape设定是什么样的,方法都不会被 auto-escaped.
1 |
|
如果要对函数强制转义输出,只需通过管道将其传递到转义筛选器。
1 |
|
逻辑标签
Swig包含一些基本的可选的代码块,叫做标签,使用标签可以更好的控制模板的渲染输出。标签示例如下:
1 |
|
其中
{ % endif % }
和{ % endfor % }
是结束标签,用于表明代码块的的结束。
1 |
|
更多关于tag的信息,请查看官方文档,这里挑几个重点的经常见到的说说。
block标签
用于声明一个代码块,继承的子模板中间可以改写或者拓展父模板中同名的代码块。也可查看#继承
1 |
|
if-else-endif
和if-elseif-endif
标签
这个与java中的if功能类似,做条件判断时使用。满足条件的内容将会被输出。
1 |
|
1 |
|
1 |
|
extends 标签
使当前的模板继承一个父模板,这个标签必须位于模板的最前面。其他查看#继承
1 |
|
for标签
用于遍历对象和数组时使用。
参数
Name | Type | 可选 | Default | 描述 |
---|---|---|---|---|
key | literal |
✔ | undefined |
A shortcut to the index of the array or current key accessor. |
variable | literal |
undefined |
The current value will be assigned to this variable name temporarily. The variable will be reset upon ending the for tag. | |
in | literal |
undefined |
Literally, “in”. This token is required. | |
object | object |
undefined |
An enumerable object that will be iterated over. |
Returns
loop.index
: The current iteration of the loop (1-indexed)
loop.index0
: The current iteration of the loop (0-indexed)
loop.revindex
: The number of iterations from the end of the loop (1-indexed)
loop.revindex0
: The number of iterations from the end of the loop (0-indexed)
loop.key
: If the iterator is an object, this will be the key of the current item, otherwise it will be the same as the loop.index.
loop.first
: True if the current object is the first in the object or array.
loop.last
: True if the current object is the last in the object or array.
示例
1 |
|
1 |
|
import
Source: lib/tags/import.js
允许你引入定义在别的文件当中的宏到你当前的模板文件当中。
The import tag is specifically designed for importing macros into your template with a specific context scope. This is very useful for keeping your macros from overriding template context that is being injected by your server-side page generation.
参数
Name | Type | Optional | Default | Description |
---|---|---|---|---|
file | string orvar |
undefined |
Relative path from the current template file to the file to import macros from. | |
as | literal |
undefined |
Literally, “as”. | |
varname | literal |
undefined |
Local-accessible object name to assign the macros to. |
示例
1 |
|
注释
Comments注释在渲染时将会被忽略,他们在渲染之前就会移除,所以没人能看到你的注释,除非他们查看你的源代码。
1 |
|
空格控制符
模板中的任何空格都会被输出在最终生成的页面上。然而,你可以使用空格控制符来消除空格。
在tag的前面或者后面添加
-
来移除前面或者后面的空格。
1 |
|
注意
没有任何空格输出
继承
swig使用
extends
关键字来对模板进行继承
- layout.html
1 |
|
- index.html
1 |
|
模板
index.html
继承自layout.html
,并且改写或者实现了其中的内容。
参考:
https://www.jianshu.com/p/c5d333e6353c
http://node-swig.github.io/swig-templates/docs/api/#CacheOptionshttp://node-swig.github.io/swig-templates/docs/api/#CacheOptions
文章链接:
https://www.zywvvd.com/notes/coding/web/front-end/swig/swig-grammar/
“觉得不错的话,给点打赏吧 ୧(๑•̀⌄•́๑)૭”
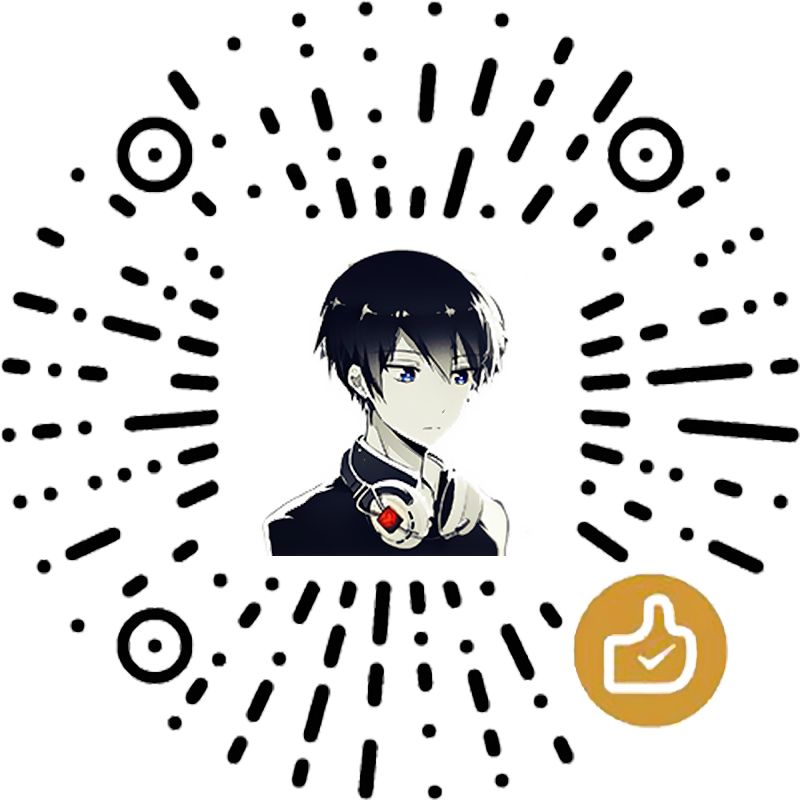
微信支付
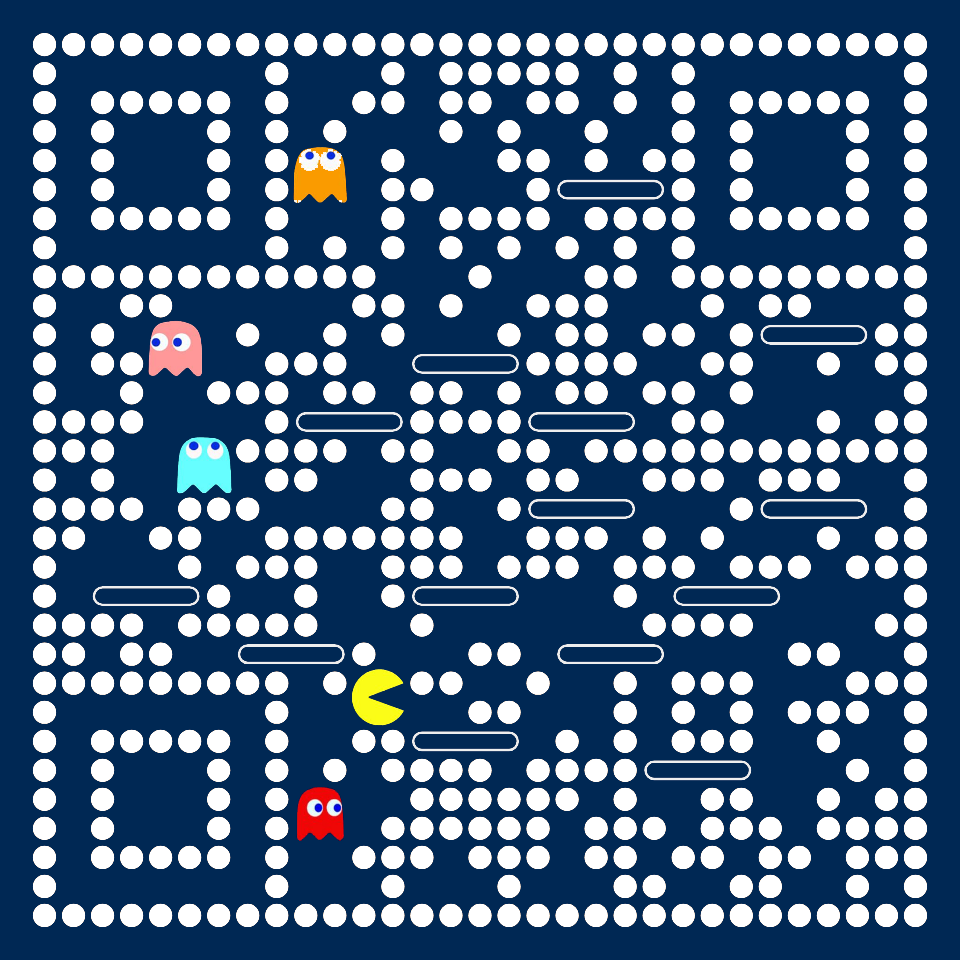
支付宝支付