本文最后更新于:2024年5月7日 下午
代码中经常会用到随机的部分,此时需要使用程序自带的伪随机数发生器,本文记录常用方法。
python 伪随机数产生方法
- python 原生 random 库
- numpy 中 random 包
random 方法
Python中的random模块用于生成随机数。下面介绍一下random模块中最常用的几个函数。
random.random()
用于生成一个0到1的随机符点数: 0 <= n < 1.0。
random.uniform(a, b)
用于生成一个在[a, b]均匀分布上的随机数。
random.randint(a, b)
用于生成一个指定范围内的整数。
random.choice(sequence)
从序列中获取一个随机元素。
random.shuffle(sequence)
用于将一个列表中的元素打乱。
random.sample(sequence, k)
从指定序列中随机(无放回)获取指定长度的片断。
numpy.random 方法
np.random.rand(d0, d1, …, dn)
产生 [d0, d1, …, dn] 维度的随机数矩阵,数据取自[0,1]均匀分布
1
np.random.rand(3,2)
np.random.randn(d0, d1, …, dn)
产生 [d0, d1, …, dn] 维度的随机数矩阵,数据取自标准正态分布
np.random.randint(low, high, size)
产生半开半闭区间 [low, high)中的随机整数,返回 size 个
np.random.choice(a, size, replace, p)
a: sequence 或者 数字(表示序列)
size: 取样个数
replace: True 有放回 False 无放回
p: 概率列表
1 |
|
np.random.bytes(length)
返回 length 个随机字节。
1 |
|
np.random.permutation(X)
返回一个随机排列
1 |
|
np.random.shuffle(X)
现场修改序列,改变自身内容。
1 |
|
参考资料
文章链接:
https://www.zywvvd.com/notes/coding/python/python-random/python-random/
“觉得不错的话,给点打赏吧 ୧(๑•̀⌄•́๑)૭”
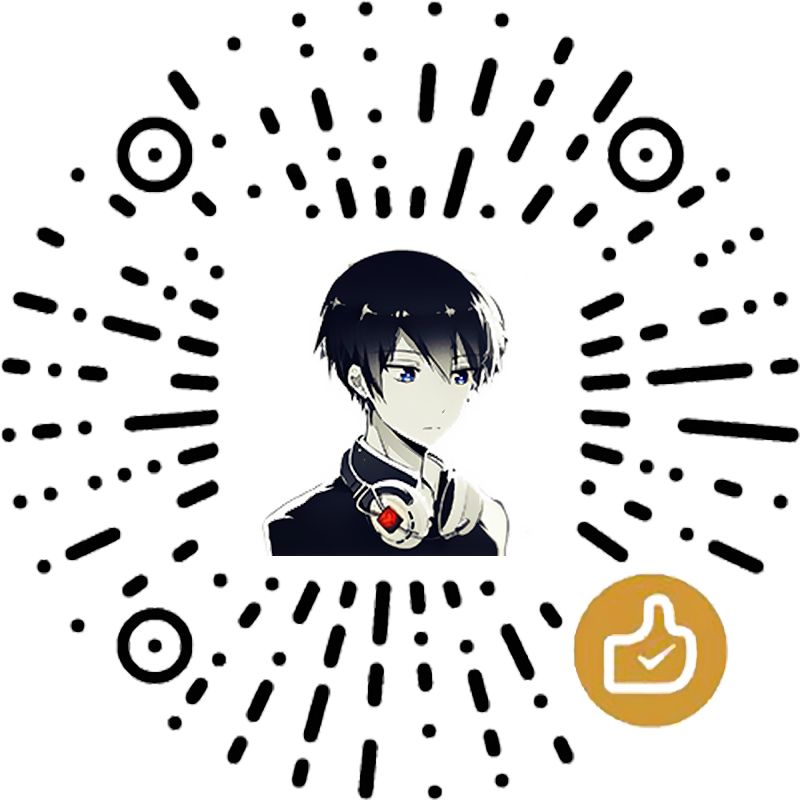
微信支付
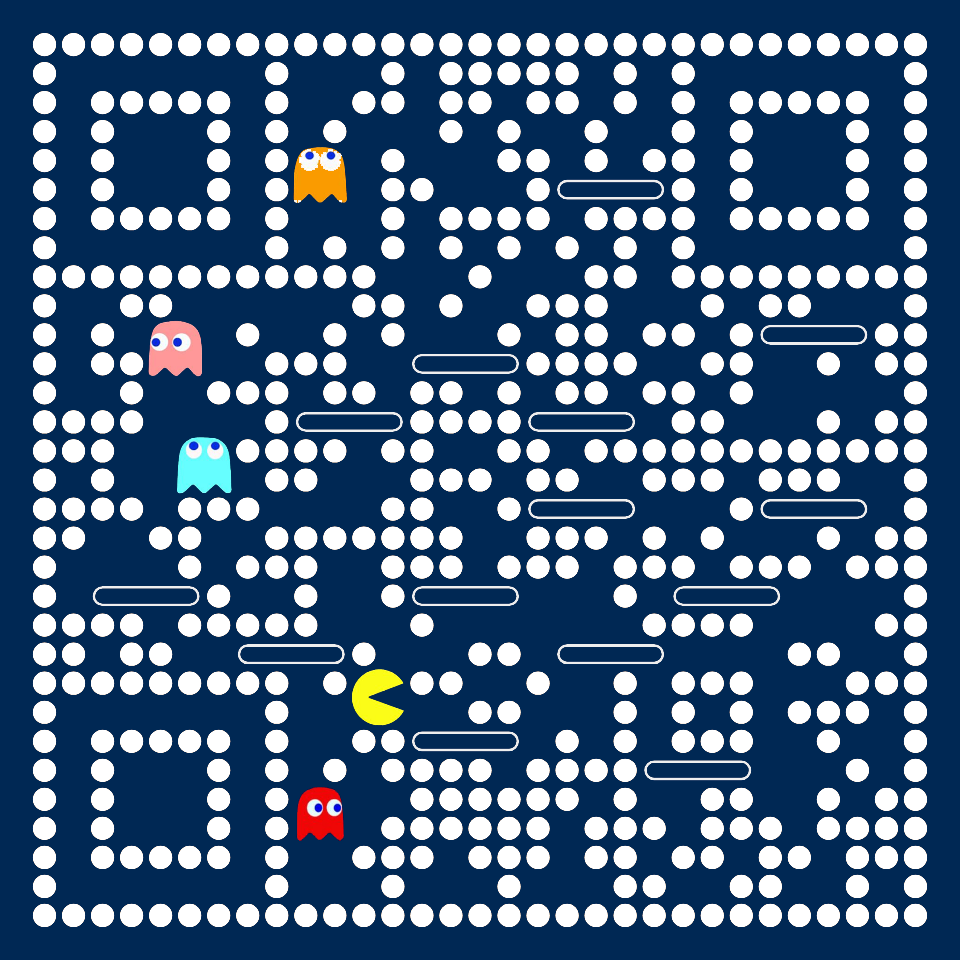
支付宝支付
Python - random 和 numpy.random 使用方法
https://www.zywvvd.com/notes/coding/python/python-random/python-random/