本文最后更新于:2023年12月5日 下午
本文记录 Node.js 脚本测试 SMTP 的两种方法。
Node.js 测试 SMTP
- node 脚本可以使用
node xxx.js
执行 - 如果有包找不到,可以使用命令安装
1 |
|
方法一
- 使用
net
包,建立连接 - 示例代码(账号使用的是测试账号,可以直接运行)
1 |
|
- 直接运行,输出结果:
1 |
|
- 登录邮箱: uipv4.zywvvd.com:33030
- 成功收到测试邮件
方法二
-
使用
nodemailer
工具 -
Nodemailer 是一个简单易用的 Node.JS 邮件发送模块(通过 SMTP,sendmail,或者 Amazon SES),支持 unicode,你可以使用任何你喜欢的字符集。
-
Github: https://github.com/nodemailer/
-
nodemailer
支持很多自定义的服务器,列表链接:https://nodemailer.com/smtp/well-known/
当前支持的服务
"126", "163", "1und1", "AOL", "DebugMail", "DynectEmail", "FastMail", "GandiMail", "Gmail", "Godaddy", "GodaddyAsia", "GodaddyEurope", "hot.ee", "Hotmail", "iCloud", "mail.ee", "Mail.ru", "Maildev", "Mailgun", "Mailjet", "Mailosaur", "Mandrill", "Naver", "OpenMailBox", "Outlook365", "Postmark", "QQ", "QQex", "SendCloud", "SendGrid", "SendinBlue", "SendPulse", "SES", "SES-US-EAST-1", "SES-US-WEST-2", "SES-EU-WEST-1", "Sparkpost", "Yahoo", "Yandex", "Zoho", "qiye.aliyun"- 示例代码(以QQ为例,需要去QQ邮箱开启 IMAP/SMTP 服务并申请授权码)
1 |
|
- 成功收到邮件:
参考资料
文章链接:
https://www.zywvvd.com/notes/coding/node-js/node-js-smtp/node-smtp/
“觉得不错的话,给点打赏吧 ୧(๑•̀⌄•́๑)૭”
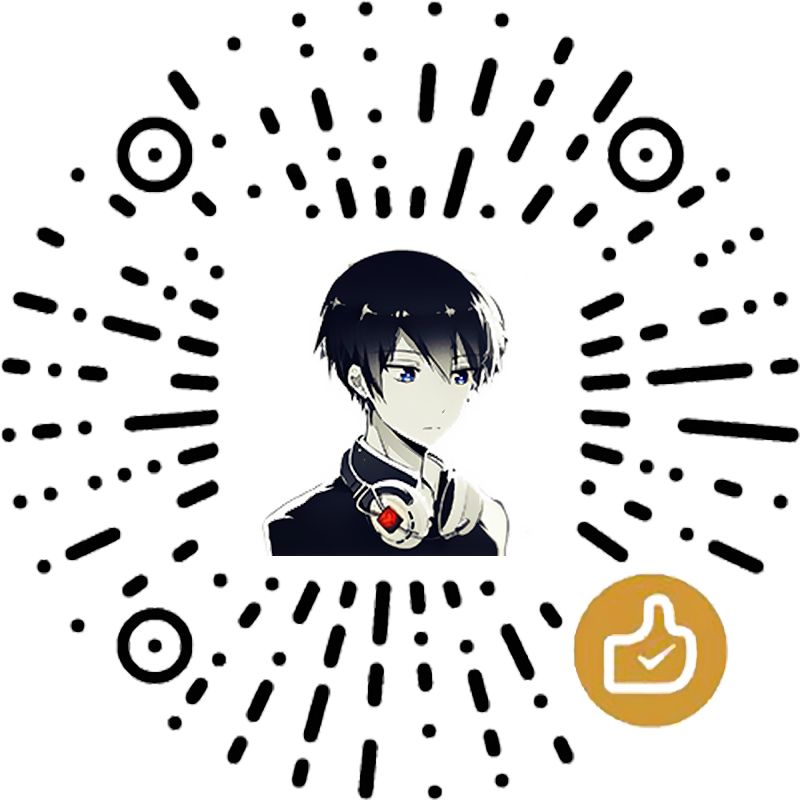
微信支付
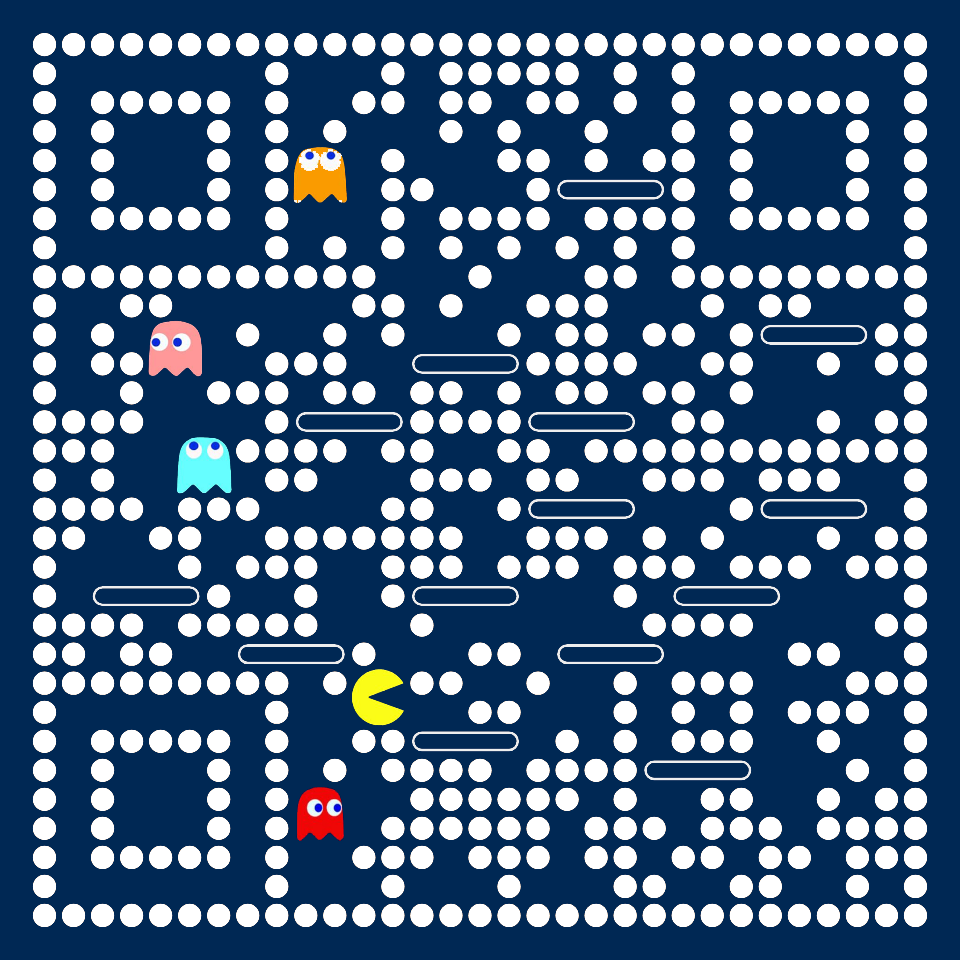
支付宝支付
Node.js 测试 SMTP 服务
https://www.zywvvd.com/notes/coding/node-js/node-js-smtp/node-smtp/