本文最后更新于:2024年5月7日 下午
本文记录 node.js 最基本的语法。
数据类型
Node.js有一些核心类型:number,boolean,string,object、undefined 和 function。
可以使用 typeof
查看数据类型
number
数字
1 |
|
boolean
true 和 false
1 |
|
string
字符串
1 |
|
object
node.js 对象,由 {} 定义 或 new Object();
1 |
|
undefined
意味着尚未设置或根本不存在
1 |
|
function
函数类型
1 |
|
运算符表达式
算术运算符
- 加+,减-,乘*,除 /,括号();
1 |
|
比较运算符
- 等于==,小于等于<=,大于等于 >=,不等于 !=
1 |
|
逻辑运算符
- 与&&,或 || 非 !
1 |
|
字符串相关运算符
1 |
|
简约表达式
- +=, -=, *=, /=
1 |
|
- 自增++,自减 –
1 |
|
条件语句
if 语句
- 单个条件
语法
1 |
|
示例
1 |
|
- 默认否
语法
1 |
|
示例
1 |
|
- 多个条件
语法
1 |
|
示例
1 |
|
switch 语句
语法
1 |
|
示例
1 |
|
循环语句
while 语句
语法
1 |
|
示例
1 |
|
for 语句
语法
1 |
|
示例
1 |
|
do while 语句
语法
1 |
|
示例
1 |
|
循环控制语句
continue
终止当前循环体的本次循环
1 |
|
break
结束最近一个循环体所有的循环
1 |
|
字符串常用操作
indexOf
要查找具有另一个字符串的字符串,请使用indexOf
函数:
1 |
|
substr和splice
要从字符串中提取子字符串,请使用substr
或splice
函数。
substr
获取要提取的字符串的起始索引和长度。splice
取起始索引和结束索引:
1 |
|
Split
要将字符串拆分为子字符串,请使用split函数并获取数组作为结果:
1 |
|
trim
trim函数从字符串的开头和结尾删除空格:
1 |
|
replace
replace替换字符串
1 |
|
String 对象属性
属性 | 描述 |
---|---|
constructor | 对创建该对象的函数的引用 |
length | 字符串的长度 |
prototype | 允许您向对象添加属性和方法 |
String 对象方法
方法 | 描述 |
---|---|
charAt() | 返回在指定位置的字符。 |
charCodeAt() | 返回在指定的位置的字符的 Unicode 编码。 |
concat() | 连接两个或更多字符串,并返回新的字符串。 |
fromCharCode() | 将 Unicode 编码转为字符。 |
indexOf() | 返回某个指定的字符串值在字符串中首次出现的位置。 |
lastIndexOf() | 从后向前搜索字符串。 |
match() | 查找找到一个或多个正则表达式的匹配。 |
replace() | 在字符串中查找匹配的子串, 并替换与正则表达式匹配的子串。 |
search() | 查找与正则表达式相匹配的值。 |
slice() | 提取字符串的片断,并在新的字符串中返回被提取的部分。 |
split() | 把字符串分割为字符串数组。 |
substr() | 从起始索引号提取字符串中指定数目的字符。 |
substring() | 提取字符串中两个指定的索引号之间的字符。 |
toLowerCase() | 把字符串转换为小写。 |
toUpperCase() | 把字符串转换为大写。 |
trim() | 去除字符串两边的空白 |
valueOf() | 返回某个字符串对象的原始值。 |
String HTML 包装方法
HTML 包装方法返回加入了适当HTML标签的字符串。
方法 | 描述 |
---|---|
anchor() | 创建 HTML 锚。 |
big() | 用大号字体显示字符串。 |
blink() | 显示闪动字符串。 |
bold() | 使用粗体显示字符串。 |
fixed() | 以打字机文本显示字符串。 |
fontcolor() | 使用指定的颜色来显示字符串。 |
fontsize() | 使用指定的尺寸来显示字符串。 |
italics() | 使用斜体显示字符串。 |
link() | 将字符串显示为链接。 |
small() | 使用小字号来显示字符串。 |
strike() | 用于显示加删除线的字符串。 |
sub() | 把字符串显示为下标。 |
sup() | 把字符串显示为上标。 |
Math 常用功能
完整介绍:https://www.w3cschool.cn/jsref/jsref-obj-math.html
Math 属性
属性 | 描述 |
---|---|
E | 返回算术常量 e,即自然对数的底数(约等于2.718)。 |
LN2 | 返回 2 的自然对数(约等于0.693)。 |
LN10 | 返回 10 的自然对数(约等于2.302)。 |
LOG2E | 返回以 2 为底的 e 的对数(约等于 1.414)。 |
LOG10E | 返回以 10 为底的 e 的对数(约等于0.434)。 |
PI | 返回圆周率(约等于3.14159)。 |
SQRT1_2 | 返回返回 2 的平方根的倒数(约等于 0.707)。 |
SQRT2 | 返回 2 的平方根(约等于 1.414)。 |
Math 方法
方法 | 描述 |
---|---|
abs(x) | 返回 x 的绝对值。 |
acos(x) | 返回 x 的反余弦值。 |
asin(x) | 返回 x 的反正弦值。 |
atan(x) | 以介于 -PI/2 与 PI/2 弧度之间的数值来返回 x 的反正切值。 |
atan2(y,x) | 返回从 x 轴到点 (x,y) 的角度(介于 -PI/2 与 PI/2 弧度之间)。 |
ceil(x) | 对数进行上舍入。 |
cos(x) | 返回数的余弦。 |
exp(x) | 返回 Ex 的指数。 |
floor(x) | 对 x 进行下舍入。 |
log(x) | 返回数的自然对数(底为e)。 |
max(x,y,z,…,n) | 返回 x,y,z,…,n 中的最高值。 |
min(x,y,z,…,n) | 返回 x,y,z,…,n中的最低值。 |
pow(x,y) | 返回 x 的 y 次幂。 |
random() | 返回 0 ~ 1 之间的随机数。 |
round(x) | 把数四舍五入为最接近的整数。 |
sin(x) | 返回数的正弦。 |
sqrt(x) | 返回数的平方根。 |
tan(x) | 返回角的正切。 |
垃圾回收
- 一个对象如果没有任何引用变量指向这个对象会被判定为垃圾对象;
- 在特定的时期js解释引擎会回收复杂对象的内存;
- 垃圾回收
- 没有任何变量,保存了这个对象的引用;
- 在特定的时候,由js解释引擎(v8)特定的时期回收
参考资料
文章链接:
https://www.zywvvd.com/notes/coding/node-js/node-js-grammer/node-js-grammer/
“觉得不错的话,给点打赏吧 ୧(๑•̀⌄•́๑)૭”
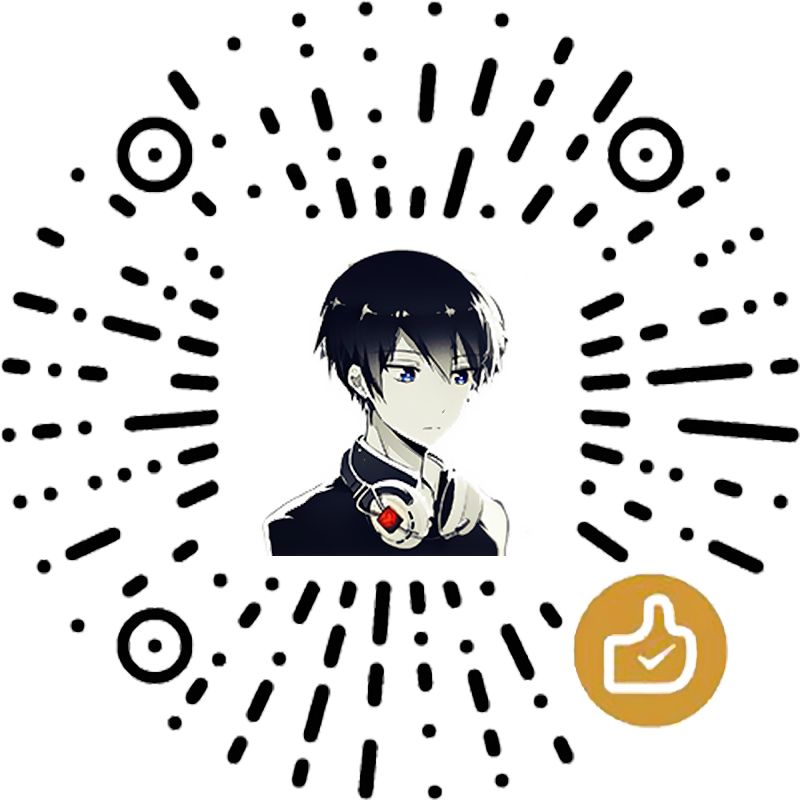
微信支付
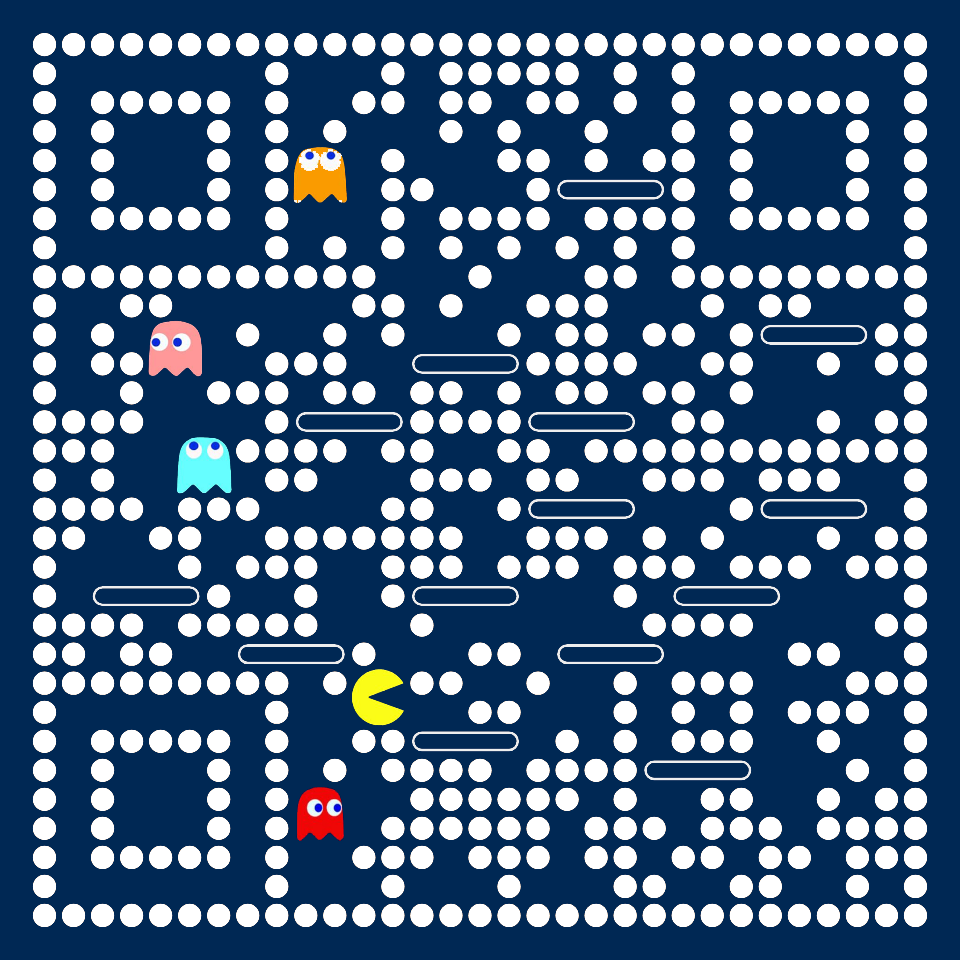
支付宝支付