本文最后更新于:2023年12月5日 下午
JSON是一种纯字符串形式的数据,它本身不提供任何方法(函数),非常适合在网络中进行传输,C++ 中操作 JSON 数据主要使用 Jsoncpp 库,本文记录使用方法。
简介
- JSON 是一种轻量级的数据交换格式,它可以表示数字、字符串、有序的值序列和名称/值对的集合。
- JsonCpp 是一个 C++ 库,它允许操作 JSON 值,包括与字符串之间的序列化和反序列化。它还可以在非序列化/序列化步骤中保留现有的注释,使其成为存储用户输入文件的方便格式。
- github 仓库:https://github.com/open-source-parsers/jsoncpp
- 官方文档:http://open-source-parsers.github.io/jsoncpp-docs/doxygen/index.html
使用配置
下载源码
- 在 github 仓库中下载源码
编译
需要安装 Cmake, VS 环境
- 我们自己编译需要用到的库,这就用到了 CMake 的 Gui 工具
- 运行
cmake-gui
选择源码文件夹和目标文件夹(build2) - 点击
configure
,在弹出的页面中,第一项会默认选择 VS,第二项选择x64
,其它不用选
- 点击
finish
- CMake 准备好后点击
Generate
-
之后选择
Open Project
打开 VS -
选择
release
或者debug
版本,在右侧解决方案中,右键ALL BUILD
, 选择重新生成
- 运行成功后即完成了源码的编译
环境配置
-
在我们已经建立的 C++ 工程中使用 JsonCpp 库需要配置相应环境
-
选择和 JsonCpp 相同的编译平台,以
Debug X64
为例
- 包含目录中加入源码的
include
文件夹
- 附加库目录加入编译生成的
lib/Debug
(如果是Release
就换成Release
) 文件夹
- 在附加依赖项中加入
jsoncpp.lib
文件
- 此外,还需要告诉系统我们刚刚编译好的
dll
文件位置,可以选择将文件直接放到项目目录中 - 我们的
dll
文件位置在build/bin/Debug/jsoncpp.dll
,将其拷贝到项目中即可
JsonCpp使用
官方文档有详细的应用,
引入工程
- 在代码中引入
json.h
文件,即可使用 JsonCpp 的功能
1 |
|
读取 Json
- 读 json 文件的思路是:
- 创建
ifstream
对象导入文件字符流 - 建立
Json::Value
对象用于接收 json 信息 - 使用 Json::Reader 对象的
parse
方法将字符流解析到 Value 对象中
- 创建
- 示例 json 文件:
1 |
|
- 示例代码:
1 |
|
可以通过此代码获取数据
560
写入 Json
- 思路和读取的类似,顺序反过来:
- 构造需要写到磁盘的
Json::Value
对象,加入需要保存的数据 - 用
Json::StyledWriter
将对象导出成字符串 - 将字符串通过
ofstream
写入本地文件
- 构造需要写到磁盘的
- 示例代码
1 |
|
- 输出 json 文件
1 |
|
参考资料
- https://github.com/open-source-parsers/jsoncpp
- http://open-source-parsers.github.io/jsoncpp-docs/doxygen/index.html
- https://blog.csdn.net/iemsadw/article/details/120311857
文章链接:
https://www.zywvvd.com/notes/coding/cpp/jsoncpp/jsoncpp/
“觉得不错的话,给点打赏吧 ୧(๑•̀⌄•́๑)૭”
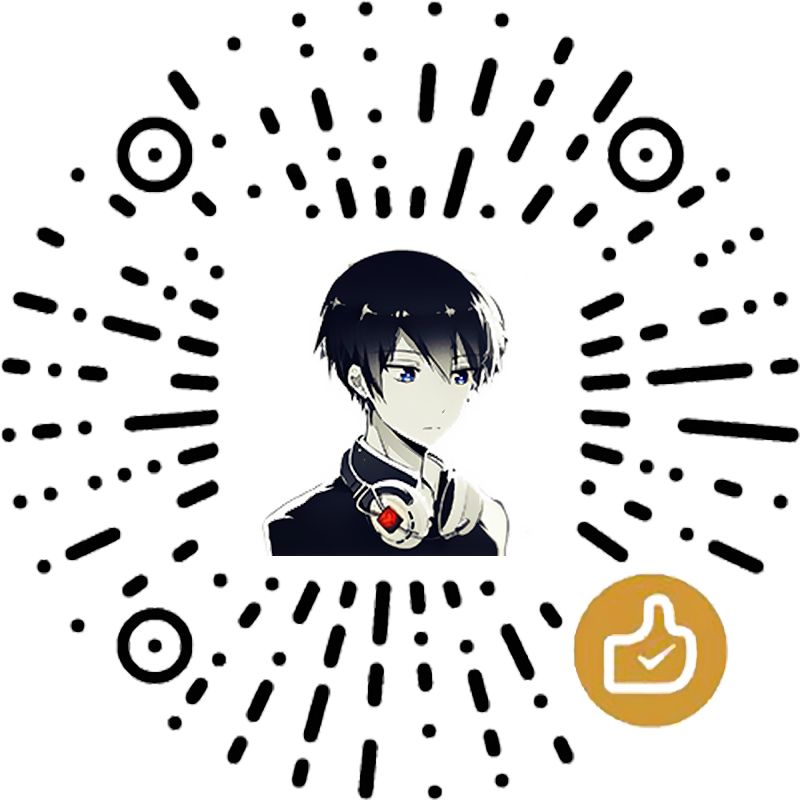
微信支付
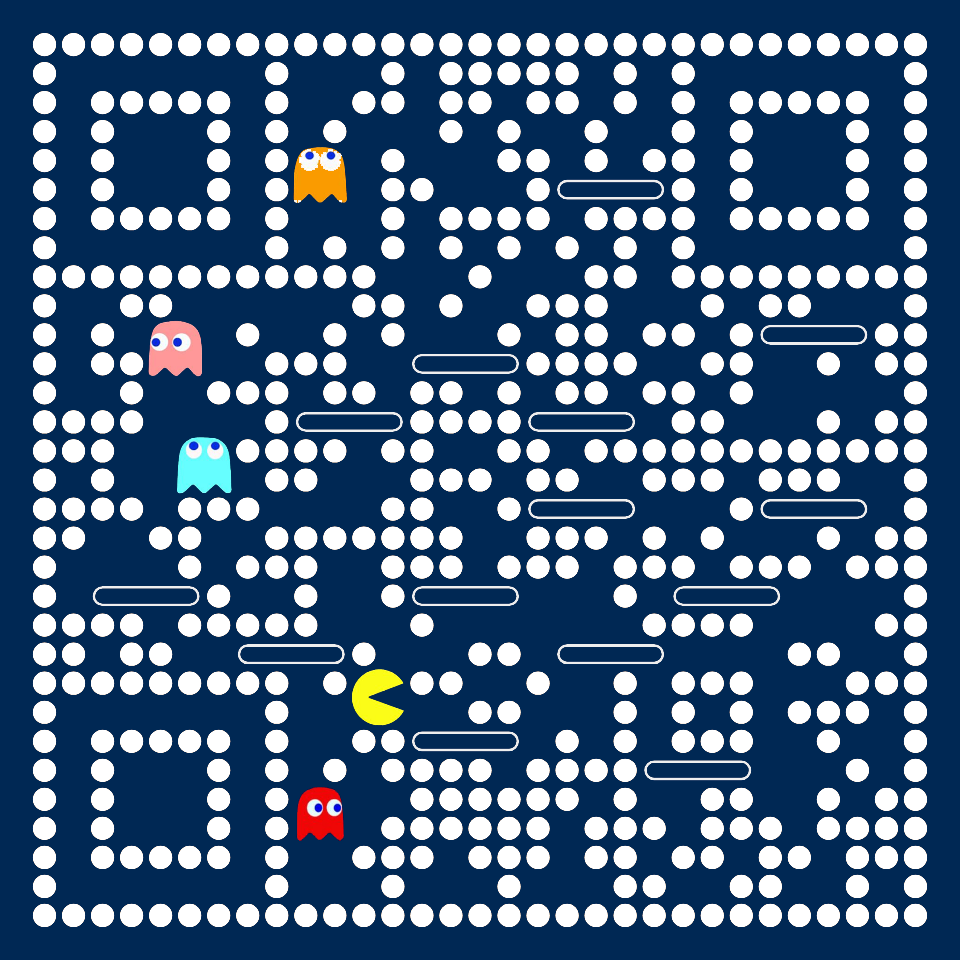
支付宝支付
Visual Studio 配置 C++ JsonCpp 使用环境
https://www.zywvvd.com/notes/coding/cpp/jsoncpp/jsoncpp/